5. Generate High-Res 2D Classes#
This example covers how to generate full-resolution 2D Class images for publication. The results are saved as PNG and PDF format.
First initialize the CryoSPARC
client.
from cryosparc.tools import CryoSPARC
cs = CryoSPARC(host="cryoem0.sbi", base_port=40000)
assert cs.test_connection()
Connection succeeded to CryoSPARC command_core at http://cryoem0.sbi:40002
Connection succeeded to CryoSPARC command_vis at http://cryoem0.sbi:40003
Connection succeeded to CryoSPARC command_rtp at http://cryoem0.sbi:40005
Load the templates output from the Select 2D job with the final selected templates.
project = cs.find_project("P251")
job = project.find_job("J10")
templates_selected = job.load_output("templates_selected", slots=["blob"])
This job has 10 selected templates. Each template is stored in a MRC file created by the 2D Classification job. The templates_selected
contains the location of these. Load all the unique paths (organized by path in a Python dictionary).
unique_mrc_paths = set(templates_selected["blob/path"])
all_templates_blobs = {path: project.download_mrc(path)[1] for path in unique_mrc_paths}
Use matplotlib to create a 5x2 grid to display these templates. Load the MRC template image data for each template. Particles for this dataset were extracted at a box size of 386px. Use a DPI slightly higher than this to allow for margins.
%matplotlib inline
from pathlib import Path
import matplotlib.pyplot as plt
N = templates_selected["blob/shape"][0][0]
scale = 100 / templates_selected["blob/psize_A"][0] # 100 Å in pixels
fig, axes = plt.subplots(3, 5, figsize=(5, 3), dpi=400)
plt.margins(x=0, y=0)
for i, template in enumerate(templates_selected.rows()):
path = template["blob/path"]
index = template["blob/idx"]
blob = all_templates_blobs[path][index]
ax = axes[i // 5, i % 5]
ax.axis("off")
ax.imshow(blob, cmap="gray", origin="lower")
if i % 5 > 0:
continue
# Plot scale bar
ax.plot([N // 7, N // 7], [N / 2 + scale / 2, N / 2 - scale / 2], color="white")
ax.text(
N // 8,
N / 2,
"100 Å",
rotation=90,
horizontalalignment="right",
verticalalignment="center",
fontsize=6,
color="white",
)
fig.tight_layout(pad=0, h_pad=0.4, w_pad=0.4)
fig.savefig(Path.home() / "class2d.png", bbox_inches="tight", pad_inches=0)
fig.savefig(Path.home() / "class2d.pdf", bbox_inches="tight", pad_inches=0)
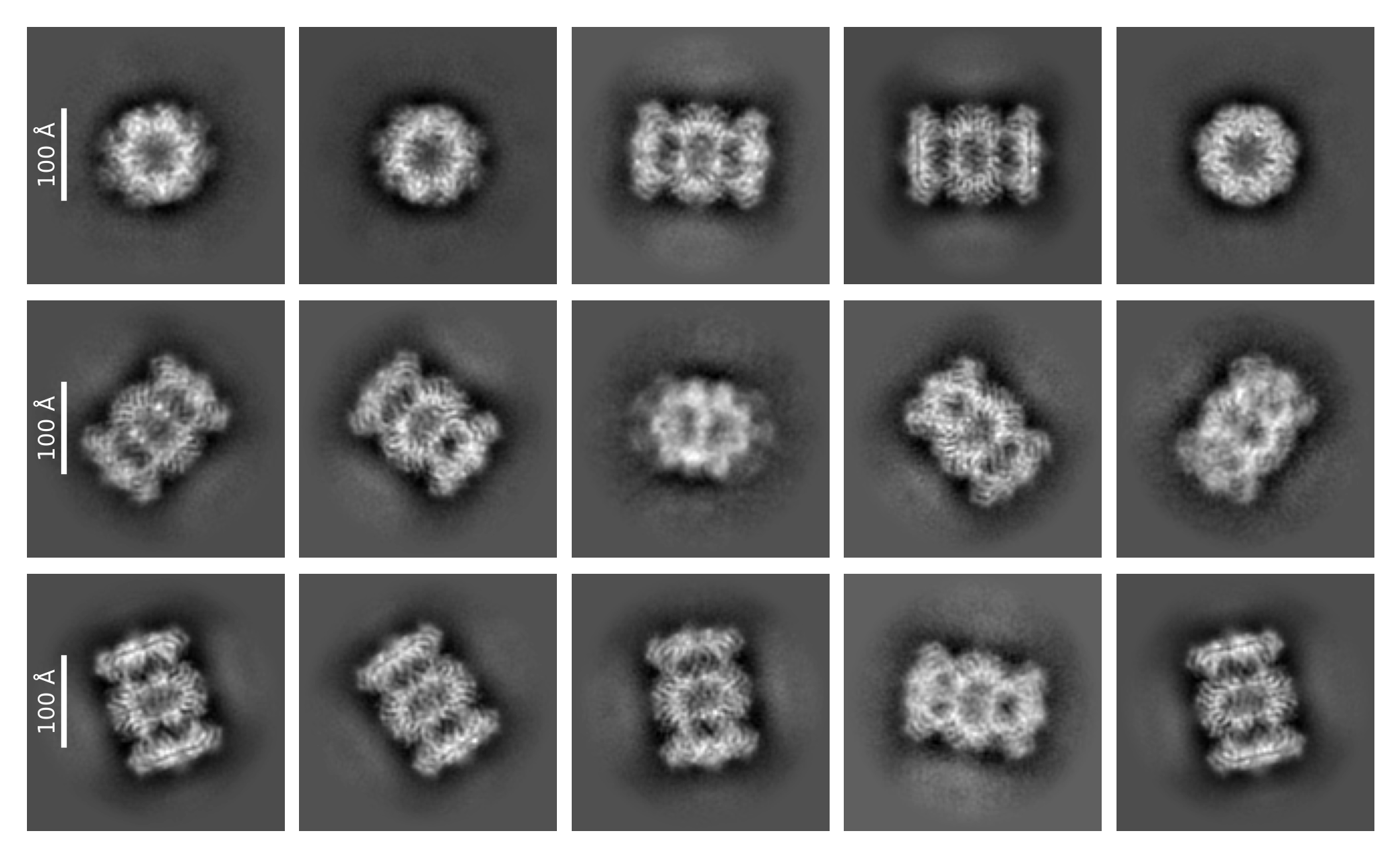
The resulting output may also be be appended to the job’s event log.
job.log_plot(fig, text="2D Classes", raw_data="Hello,Templates", raw_data_format="txt")
'64f9f9daa567446896198d9f'